Module 1
index
Basics
C language comments :
// this is single line comment
/*
This is multiple line comment
You can writ anything hear... compilier just ignore this..
*/
Basic rules:
- Every C program requries a main() function (Use of more then one main() is illegal). The place main is where the program execution begins.
- The execution of a function begins at the opening brace of the function and ends at the corresponding closing brance.
- C programs are written in lowercase letters, Howeve, uppercase letters are usec for symbolic names and output strings.
- Every program statement in a C language must end with a semicolon.
- All Variables must be declared for their types before they are used in the program.
- We must make sure to include header files using #include directive when the program refers to special names and functions that it does not degine.
Basic Structuer Of C language
- The documentation section consists of a seat of comment lines giveing the name of th program, the author and other details, which the programmer would like to use later.
- The definition section defines all symbolic constants.
- There are some variables that are used in more than one function. Such variables are called global variables and are declares in the gloabal declariton section that is outside of all the functions. This section alse declares all the user-defined functions.
- Every C program must have one main() function section. This section contains two parts, declaration part and executable part. The declaration part declares all the variables used in the executable part. There is at least one statement in the executable part. These two parts must appear between the opening and the closing brance and ends at the closing brance. The closing brace of the main function section is the logical end of the program. All statements in the declaration and executable parts end with a semicolon(;).
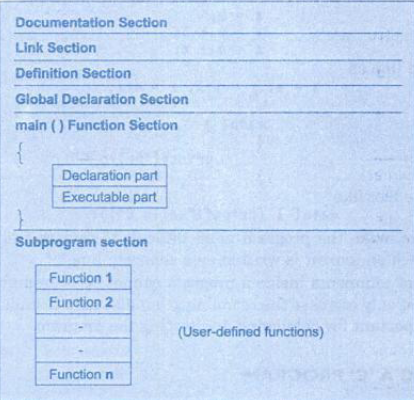
Compiling and Linking
GCC Compiler:
- One of the most popular C compiler is the GCC compiler. which is supplied with Linux but is abailable for many other platforms are well. Using this compiler is similar to using the treditional UNIX cc compiler.
Compiling:
- Let us assume that the source program has been created in a file named kaushal.c. Now the program is ready for compilation.
- The compilation command to achieve this task under UNIX is:
cc kaushal.c
- The source program instruction are now translated into a form that is suitable for execution by the computer. The translation is done after examining each instruction for its correctness. If every thing is alright, the compileation proceeds silently and the translated program is stored on another file with the name kaushal.o. This program is knowan as object code.
Linking:
- Linking is the process of putting together other program files and functions that are requierd by the program.
- For example, if the program is using exp() function, then the object code of this function should be brought from the math library of the system and linked to the main program.
- Under UNIX, The linking is automatically done when the cc command is used.
- The compiled and linked program is called the executable object code and is stored automatically in another file named
a.out
.
- Note windows genrate
a.exe
insted of a.out
, both work same.
- Note that the linker always assigns the same name a.out. When we compile another program, this file will be overwritten by the executable object code of the mew program.
- If we want to prevent from happening, we should rename the file immediatelly by using the command.
- we may also achieve this by spacifying an option in the cc command as follows:
- This will store the executable object code in the file name and prevernt the old file
a.out
from being destroyed.
Q&A
- Q: What does GCC stand for?
- A: GCC originally stood for “GNU C Compiler”. It now stand for “GNU Compiler Collection.” because the current version of GCC compiles program written in a variety of languages. including Ada, C, C++, Fortran, Java, and objective-C.
- Q: What’s the big deal about GCC, anyway?
- A: GCC is significiant for many reasons.
Hello World
printf
- This file as a startup for entering in c language
- creating first file using .c extanstion
#include <stdio.h>
int main()
{
printf("hello world\n");
return 0;
}
- printf is used for printing text and outputs.
Basic calculation with c
int a, b;
a = 34;
b = 6;
printf("a - b = %d\n", a-b);
printf("a + b = %d\n", a+b);
printf("a * b = %d\n", a*b);
printf("a / b = %d\n", a/b);
- try with yourself by changing a and b values
- above code must in
int main(){ // code section}
Exercise with answer 🔬
Attachments 📎
Get value from user
- For gatting value from user we use scanf from stdio.h library.
- Scanf creat so simple to get value from user.
- For gatting value from user use below steps
- define a variable
- use scanf command
- use printf command for showing result.
#include<stdio.h>
int main()
{
int a; // define a
printf("Enter first Value\n");
scanf("%d", &a); // get value from user
printf("Our value of a is %d", a); // output
return 0;
}
Exercise with answer 🔬
Attachments 📎
Multiple Source File
- To compile and link multiple source program files, we must append all the files name to the cc command.
cc filename-1.c ... filename-n.c
- This file will be seprately compiled into object file called
filename-i.o
and then linked to produce and executable program file a.out.
- It is also possible to compile each file seprately and link them later. For example, the commands
cc -c mod1.c
cc -c mod2.c
- Will compile the source files mod1.c and mod2.c objects files mod1.o and mod2.o. They can be linked together by the command
- we may also combine the source files and object file as follows:
- Only mod1.c is compiled and then linked with the object file mod2.o. This approch is useful whenn one of the multiple source files need to be changed and recompiled or an alrady existing object files is to be used along with the program to be compiled.
Exp:
- We need to creat two c files, main.c and multipli.c
main.c:
#include <stdio.h>
int multiplication();
int main(int argc, char const *argv[])
{
int x;
printf("enter the value for multiplication: ");
scanf("%d", &x);
multiplication(x);
return 0;
}
multipli.c:
#include <stdio.h>
#define MAX 10
int multiplication(int x)
{
int i = 1;
while (i <= MAX)
{
printf("%3d x %2d = %5d \n", x, i, x*i);
i++;
}
}
- Now we compile both using following command:
- compile and linking procces give as single exicutable file a.out which is combo of both c files.
Attachments 📎
Variables
A variable is a name of the memory location. It is used to store data. Its value can be changed, and it can be reused many times.
It is a way to represent memory location through symbol so that it can be easily identified.
The example of declaring the variable is given below:
int a;
float b;
char c;
// assigning values method 1
// first declare then assign
int a;
a = 44;
// method 2
// declare and asign at same time
int a = 44;
float b = 43.33;
float c = 48.44f; // only float support f after value, This helps to know this is flot value.
// output are always same with or without f.
Hear, a, b, c are variables. The int, float, char are the data types.
Rules for defining variables
- A variable can have alphabets, digits, and underscore.
- A variable name can start with the alphabet, and underscore only. It can’t start with a digit.
- No whitespace is allowed within the variable name.
- A variable name must not be any reserved word or keyword, e.g. int, float, etc.
Types of Variables in C
- local variable
- global variable
- static variable
- automatic variable
- external variable
External variable
We can share a variable in multiple C source files by using an external variable. To declare an external variable, you need to use extern keyword.
ex:
myfile.h
extern int x=10;//external variable (also global)
program1.c
#include "myfile.h"
#include <stdio.h>
void printValue(){
printf("Global variable: %d", global_variable);
}
Attachments 📎
Operators
An Operators is a symbol used to perform operations in given programming language.
Types of operators
- Arithmetic operators
- Relation Operators
- Logical Operators
- Bit wise operators
- Assignment operators
- Miscellaneous
Arithmetic operators
- This operators are basic operators for day to day life.
Operators |
Description |
+ |
Addition |
- |
Subtraction |
* |
Multiplication |
/ |
Division |
% |
modules |
Relation operator
Operators |
Description |
== |
Is equal to |
!= |
Is not equal to |
> |
Greater than |
< |
Less than |
>= |
Greater than |
<= |
Less than or equal to |
Note: mostly this operators are used in if, else statement.
Logical operator
Operators |
Description |
Example |
&& |
Logical “AND” operator, both the operands are non-zero then condition is true. |
a&&b |
∥ |
Logical “OR” operator, if any of these two operands is none-zero, Then condition become true. |
a∥b |
! |
Logical “NOT” operator, It is used to reverse the logical state of its operand, if condition is true. |
!a |
Bit wise operators
- Bit-wise operator are functioning on bit.
Value A |
Value B |
A&B |
A∣B |
A^B |
0 |
0 |
0 |
0 |
0 |
0 |
1 |
0 |
1 |
1 |
1 |
1 |
1 |
1 |
0 |
1 |
0 |
0 |
1 |
1 |
- XOR operator: If one value is true and second one is false, Then XOR give true value.
- other Bit wise operator
- ~ is the binary one’s complement operator
- « is the binary left shift operator
- >> is the binary right shift operator
Assignment operators
Operators |
Description |
= |
Simple assignment operator assign value from right side operands |
+= |
Add AND assignment operator. It’s adds the right operand to the left operands and assign the result to the left operands |
-= |
Subtract AND assignment operator. It subtracts the right operands from the left to the result is assignment to the left operand. |
*= |
Multiply AND assignment operator. It multiples the right operands from the left to the result is assignment to the left operand. |
/= |
Divide AND assignment operator. It divide the right operands from the left to the result is assignment to the left operand. |
Miscellaneous
Operators |
Description |
Example |
sizeof() |
Returns the size of variable. |
sizeof(a), where a is an integer, will return in’s size on that architachure. |
& |
Returns the address of variable. |
&a; return the actual address of the variable. |
* |
Pointer variable |
*a; |
?: |
Conditonal expression |
if condition is true, The value X; Otherwise value Y |
Operators PRECEDENCE IN C
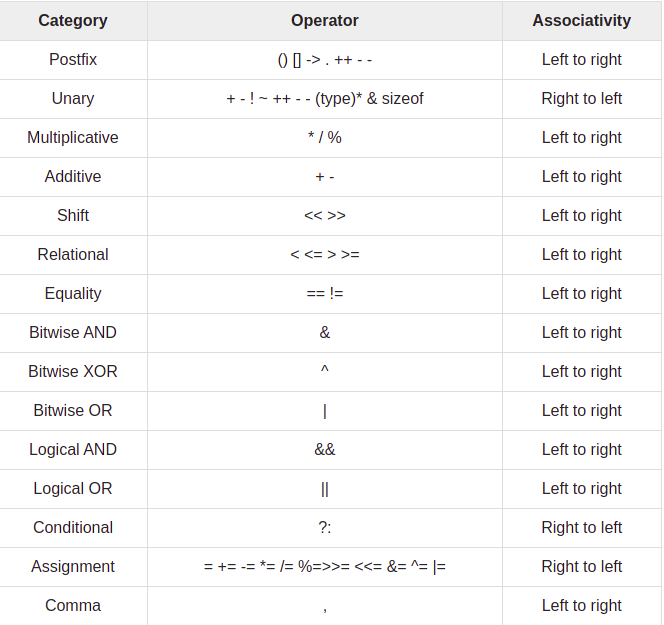
Attachments 📎
- The Format specifier is a string used in the formatted input and output functions. The format string determines the format of the input and output. The format string always starts with a ‘%’ character.
Pritf(“This is a good boy %a.bf”, var);
Will print var with b decimale in a “a” character space
- ex : ‘printf(“%5.2f”, a);`
- prints out the value of amount in floating point format. The format spaciefication %5.2f tells the compiler that the output must be in floating point, With five places in all and two places to the right of the decimal point.
format |
work |
%c |
character print |
%d |
integer print |
%f |
float print |
%l |
long print |
%lf |
double print |
%LF |
long double print |
Attachments 📎
Constant
- A constant is a value or variable that can’t be changed in the program.
- Example: 15, ‘a’, 3.4, “Name, etc..
- There are Two ways to define constant in C Program.
- Constant Keyword:
const float b = 7.499;
#define
preprocessor: #define PI 3.14
The #define Directive:
- A #define instruction defines value to a symbolic constant for use in the program. Whenever a symbolic name is encountered, the compiler substitutes the value associated with the name automatically. To change the value, we have to simply change the definition.
- A #define is a preprocessor compiler directive and not a statement. Therfore #define lines should not end with a semicolon.
- #define instrucions are usually placed at the beginnig before the main() function.
Attachments 📎
Escape sequence
- An escape sequence inn C programming, language is a sequence of characters.
- It doesn’t represent itself when used inside string literal or character.
- It is composed of two or more characters string with backslash
\
.
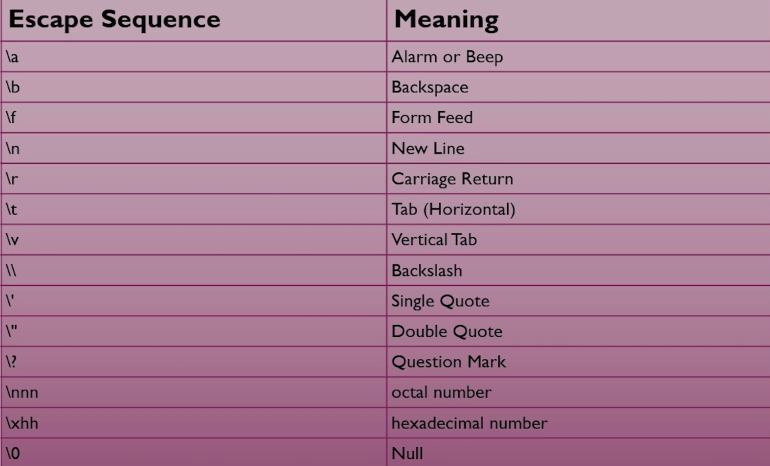
Example:
#include <stdio.h>
int main(int argc, char const *argv[])
{
printf("\t Horizontal tab\n");
return 0;
}
If else
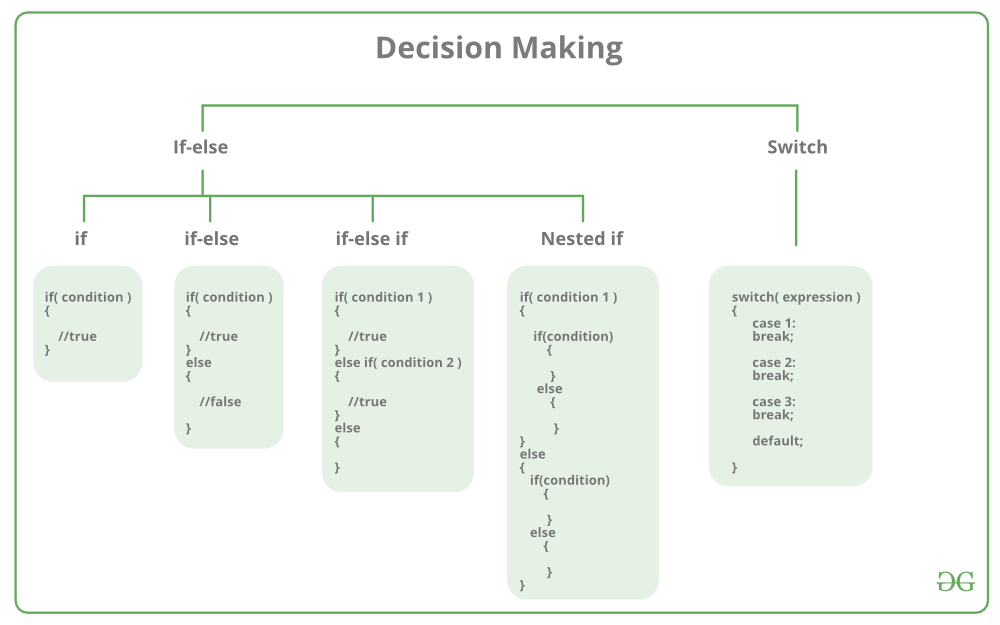
if Statement
- If statement is the most simple decision making statement. It is used to decide whether a
certain statement or block of statements will be executed or not. If a certain condition is
true then a block of statement is executed otherwise not.
Exp:
if(i == 5)
{
printf("You entered 5 as value");
}
Flow Chart:
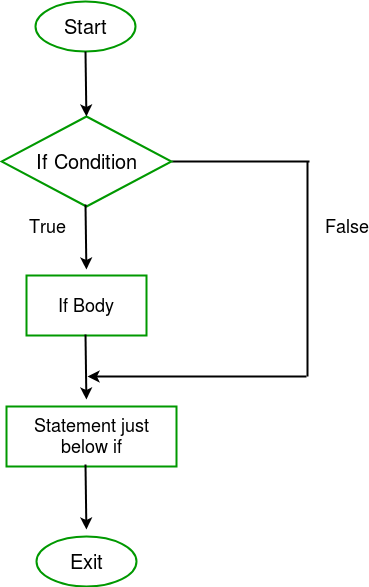
- Here, condition after evaluation will be either true or
false. C if statement accepts Boolean values – if the
value is true then it will execute the block of statements
below it otherwise not. If we do not provide the curly
braces
‘{‘
and ‘}’
after if(condition) then by default if
statement will consider the first immediately below
statement to be inside its block.
if else statement
- The if statement alone tells us that if a condition is true it will execute a block of
statements and if the condition is false it won’t. But what if we want to do something else if
the condition is false. Here comes the C else statement. We can use the else statement
with if statement to execute a block of code when the condition is false.
Exp:
if (i<j)
{
max = j;
}
else
{
max = i;
}
Flow Chart:
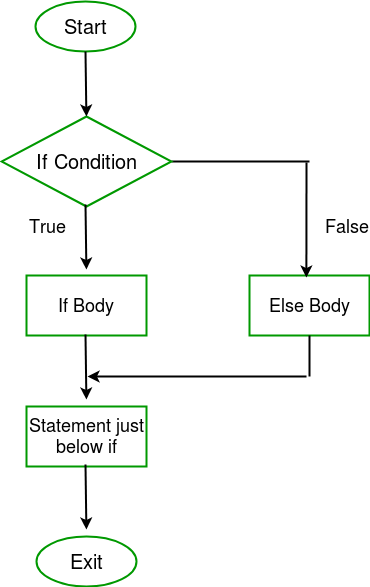
Cascaded if
- The C if statements are executed from
the top down. As soon as one of the
conditions controlling the if is true, the
statement associated with that if is
executed, and the rest of the C else-if
ladder is bypassed. If none of the
conditions are true, then the final else
statement will be executed.
Exp:
if (n<0) {
printf("n is less than 0\n");
}
else if (n ==0 ){
printf("n is equalt to 0\n");
}
else {
printf("n is greater than 0\n");
}
Flow Chart:
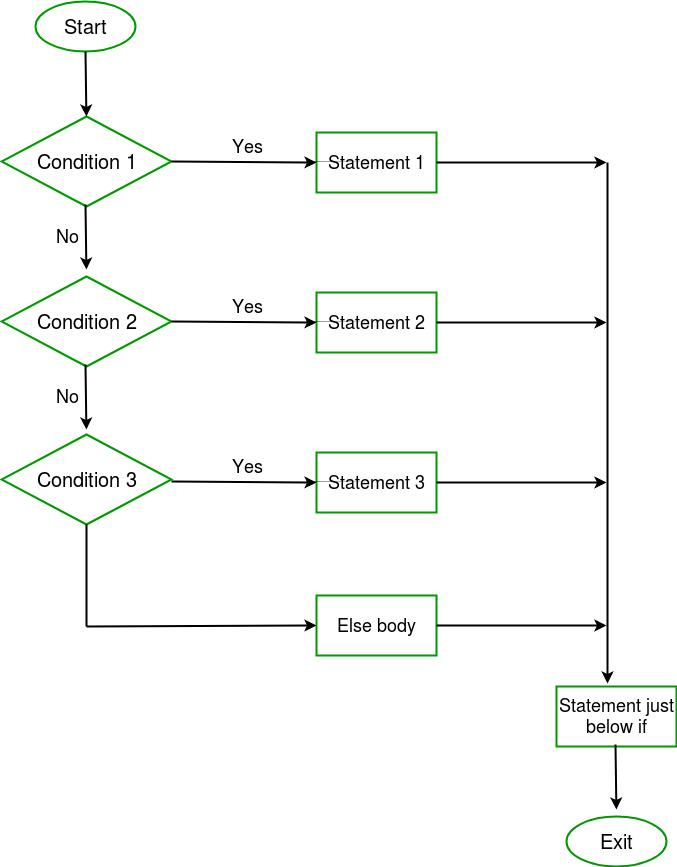
Nested if else
- A nested if in C is an if statement that is the target of another if statement. Nested if
statements means an if statement inside another if statement. Yes, both C and C++ allows
us to nested if statements within if statements, i.e., we can place an if statement inside
another if statement.
Exp:
if (i > j){
if(i > k){
max = i;
}
else{
max = k;
}
}
else
if( j > k){
max = j;
}
else {
max = k;
}
Flow Chart:
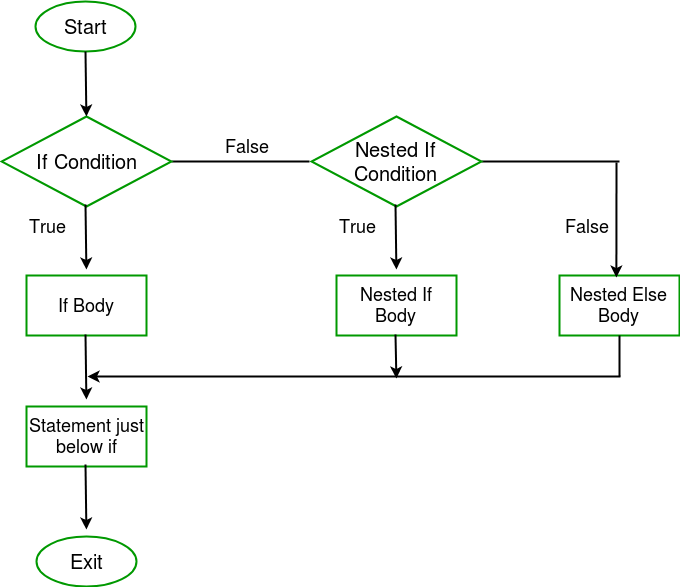
Exercise with answer 🔬
- Q. Give gift to student from there marks.
conditions :
- passed in maths and science. gift rs 45
- passed science only. gift rs 15
- passed maths only. gift rs 18.
- A. Give gift to student from there marks.c
Switch Case Statement
Rules:
- Switch expression must be int or char.
- Case value must be int or char.
- Case must come inside switch.
- Break is not a must.
Note: If break is not added, code will execute below cases also.
Types:
- Switch case without break.
- Switch case.
- Nested switch case
Syntax:
switch (n)
{
case 1: // code to be executed if n = 1;
break;
case 2: // code to be executed if n = 2;
break;
default: // code to be executed if n doesn't match any cases
Switch case without break
- if any of one statemet is truee, compiler also print below cases. when you don’t use brack statement.
ex:
switch (n)
{
case 3:
printf("You entered 3\n");
case 5:
printf("You entered 5\n");
case 8:
printf("You entered 8\n");
default:
printf("Please enter 3, 5 or 8 only.\n");
}
- In above example we not used breack statement so if any case is truee compiler also prints below casese.
- you can try above example with n = 5 value.
Switch case
ex:
switch (n)
{
case 3:
printf("You entered 3\n");
break;
case 5:
printf("You entered 5\n");
break;
case 8:
printf("You entered 8\n");
break;
default:
printf("Please enter 3, 5 or 8 only.\n");
break;
}
- We used brak statement in above example, Now if any case is true. compiler only shows that case and ignoring below cases.
Nested switch case
- Nested switch case is as same as nested if-else statement.
switch (age)
{
case 22:
printf("your age is 22\n");
switch (marks) // Nested switch
{
case 33:
printf("your maaks is 33\n");
break;
default:
printf("your marks is not 33\n");
break;
}
break;
case 17:
printf("your age is 17\n");
break;
default:
printf("Please enter valid age\n");
}
Attachments 📎